CapGemini Pseudo Code set 2
1. |
What will be the output of following code :
|
A. | i = -1 |
B. | i = 0 |
C. | i = 1 |
D. | Error : Undefined operation |
2. |
What will be the output of following code :
|
A. | 40 |
B. | 10 |
C. | 20 |
D. | Error |
3. |
What will be the output of following code :
|
A. | true |
B. | false |
C. | 0 |
D. | Error |
4. |
What will be the output of following code :
|
A. | 4 |
B. | 1 |
C. | 0 |
D. | 6 |
5. |
What will be the output of following code :
|
A. | 4 |
B. | 1 |
C. | 0 |
D. | 6 |
6. |
What will be the output of following code :
|
A. | -1 |
B. | Garbage value |
C. | 0 |
D. | Error |
7. |
What will be the output of following code :
|
A. | Two same variables can not be compared |
B. | ifelse |
C. | else |
D. | if |
8. |
What will be the output of following code :
|
A. | TRUE |
B. | FALSE |
C. | NULL |
D. | ERROR |
9. |
What will be the output of following code :
|
A. | work |
B. | not work |
C. | compiler error |
D. | runtime error |
10. |
What will be the output of following code :
|
A. | inside else block |
B. | 0 |
C. | 0inside if block |
D. | Error – If can not have print statement |
11. |
What will be the output of following code :
|
A. | 5 5 |
B. | 5 4 |
C. | 5 6 |
D. | 6 6 |
12. |
What will be the output of following code :
|
A. | 51 |
B. | 25 |
C. | 50 |
D. | None of these |
13. |
What would be output of following program ?
|
A. |
0, 1, 2 |
B. |
1, 2, 3 |
C. |
0, 2, 1 |
D. |
1, 3, 2 |
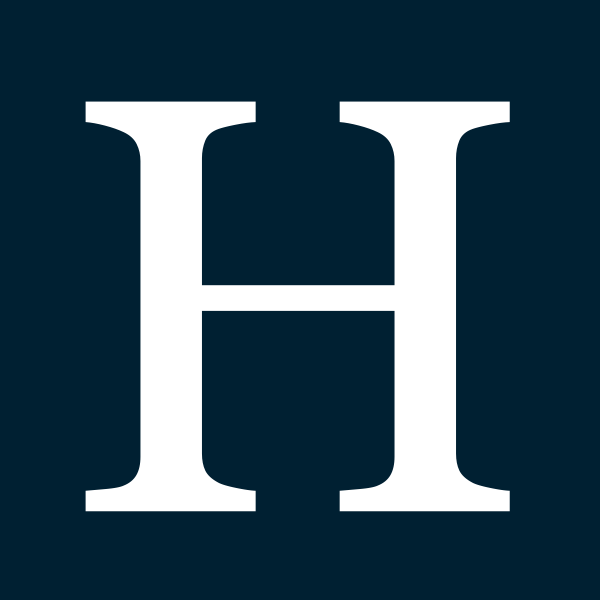
Join the community of 1 Lakh+ Developers
Create a free account and get access to tutorials, jobs, hackathons, developer events and neatly written articles.
Create a free account