CapGemini Pseudo Code set 1
1. |
What will be the output of following code :
|
A. | 8 0 |
B. | 0 0 |
C. | 16 4 |
D. | Error : Can’t Perform operation |
2. |
What is the output of given code :
|
A. | Run time Error |
B. | 32 |
C. | 64 with warning |
D. | No output |
3. |
Which of the following statements is true regarding, Auto Storage Class ? |
A. | It is used to give a reference of a global variable that is visible to all program files. |
B. | It instructs the compiler to keep a local variable in existence during the lifetime of a program. |
C. | It is the default storage class for all local variables. |
D. | It is used to define local variables that should be stored in a register. |
4. |
What will be the output of the following code :
|
A. | 984 |
B. | 98.4 |
C. | 98 |
D. | Error |
5. |
What is the output of given code (Input : 1.45):
|
A. | 1 3 |
B. | Error : Undefined condition in switch |
C. | 2 |
D. | No output |
6. |
What is the output of given code for input 134 :
|
A. | 2 |
B. | 3 |
C. | Runtime Error |
D. | None of these |
7. |
What will be output of given pseudo code for input 7 :
|
A. | 32 |
B. | 76 |
C. | 56 |
D. | 28 |
8. |
What will be output of given pseudo code for input 2 :
|
A. | 4 |
B. | 8 |
C. | 16 |
D. | Error |
9. |
What will be output of given pseudo code :
|
A. | 12 |
B. | 5 |
C. | 7 |
D. | 6 |
10. |
What will be output of given pseudo code :
|
A. | 42 36 |
B. | 36 1 |
C. | 1 1 |
D. | 1 36 |
11. |
What will be output of given pseudo code :
|
A. | 0 1 2 |
B. | 0 2 0 |
C. | 0 2 2 |
D. | Error |
12. |
integer a = 40, b = 35, c = 20, d = 10
|
A. | Differ by 80 |
B. | Same |
C. | Differ by 50 |
D. | Differ by 160 |
13. |
integer a = 60, b = 35, c = -30
|
A. | 0 and 1 |
B. | 0 and 0 |
C. | 1 and 1 |
D. | 1 and 0 |
14. |
What is the output of given code :
|
A. | 1 |
B. | 0 |
C. | Undefined Behavior due to order of evaluation can be different. |
D. | Compilation Error |
15. |
Consider the following code:
|
A. | NOT(condition2) AND NOT(condition3) |
B. | condition1 AND condition4 AND NOT(condition2) AND NOT(condition3) |
C. | condition1 AND condition2 AND condition4 |
D. | NOT(condition1) AND condition2 AND NOT(condition4) |
16. |
What will be the output of following code :
|
A. | It transposes the given matrix A |
B. | It does not alter the given matrix. |
C. | It makes the given matrix A, symmetric |
D. | None of the above. |
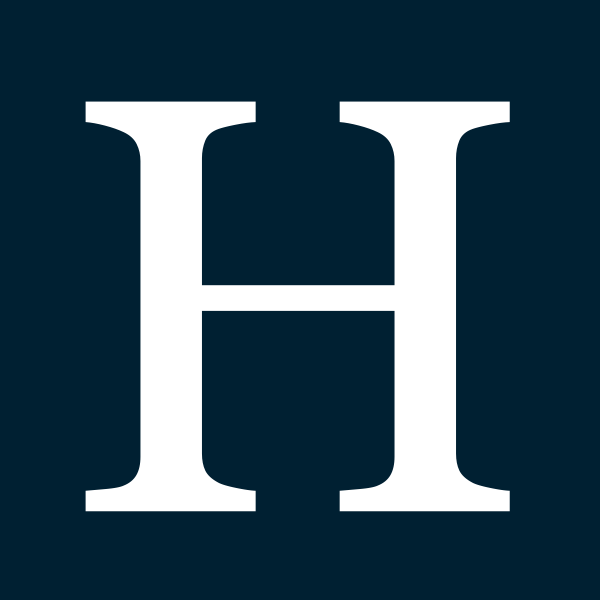
Join the community of 1 Lakh+ Developers
Create a free account and get access to tutorials, jobs, hackathons, developer events and neatly written articles.
Create a free account