C Programming variable and function declaration (set 3)
This set contains problems that require you to read any given C program and identify it's output.
1. |
What would be output of following program ?
|
A. |
0, 1, 2 |
B. |
1, 2, 3 |
C. |
0, 2, 1 |
D. |
1, 3, 2 |
2. |
Take a look at the following code:
Assume, this program is being executed on GCC compiler. What would be output of the program ? |
A. |
2 |
B. |
4 |
C. |
output will vary from one compiler to another compiler |
D. |
It will throw error. Linker Error : Undefined symbol 'x' |
3. |
What would be output of the following program ?
|
A. |
Garbage value |
B. |
It will throw an error |
C. |
0 |
D. |
98 |
4. |
Take a look at following code:
Suppose this program is being run by GCC compiler on a 64-bit machine. What would be the output ? |
A. |
4, 4, 4 |
B. |
2, 4, 2 |
C. |
2, 2, 2 |
D. |
2, 2, 4 |
5. |
What would be output of the following code:
|
A. |
Garbage values |
B. |
0, 0.000000 |
C. |
It will throw runtime error |
D. |
None of the options |
6. |
What would be output of the following code:
|
A. |
83 |
B. |
80 |
C. |
It will throw error |
D. |
None of the options |
7. |
What would be the output of the following program:
|
A. |
Compile time error |
B. |
0 |
C. |
1 |
D. |
None of the options |
8. |
What would be output of the following program:
|
A. |
7 |
B. |
8 |
C. |
7.82 |
D. |
Compile Error |
9. |
What would be the output of following of code:
|
A. |
9, 4, 4 |
B. |
Garbage values |
C. |
Compile Error |
D. |
0, 0, 0 |
10. |
What can you say about number of iterations of for loop upon execution in following code:
|
A. |
It will be executed 9 times |
B. |
It depends upon input |
C. |
It'll execute infinitely |
D. |
Compile Error |
11. |
What will be output of the following code:
|
A. |
9, 5 |
B. |
5, 9 |
C. |
Compile Error: Conflicting variable name 'i' |
D. |
5, 5 |
12. |
What would be output of the following code:
|
A. |
7, 2, garbage value |
B. |
7, 2, 0 |
C. |
7, 2, 519 |
D. |
None of the options |
13. |
What is wrong with the following code:
|
A. |
No Error |
B. |
It will print 'HackersFriend'. But it will give warning on compilation. |
C. |
Compile time error: print() is called before defined. |
D. |
None of the options |
14. |
What can you say about following code:
|
A. |
It will throw error on compilation |
B. |
It will print 0 |
C. |
It will throw error on runtime |
D. |
None of the options |
15. |
What is wrong with the following code:
|
A. |
Syntax error: Cannot create instance of struct car with 'car int myCarPrice' |
B. |
No Error |
C. |
Error in printf(): cannot print address of variable 'x' |
D. |
None of the options |
16. |
Take a look at following line of code:
What can you say about variable 'days' ? |
A. |
It is a typedef for enum weekdays. |
B. |
It is a variable of type enum weekdays. |
C. |
It will throw error |
D. |
None of the options |
17. |
What is wrong with the following code:
|
A. |
Syntax Error on line int (*x)() = foo |
B. |
Compile Error: foo undeclared. |
C. |
No Error |
D. |
None of the options |
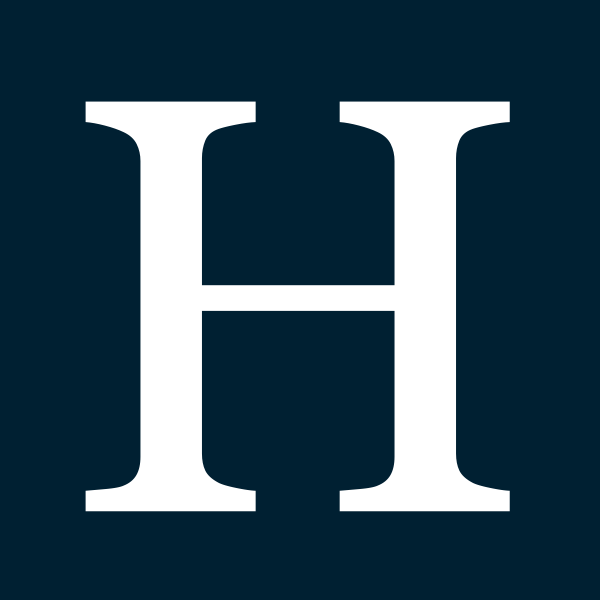
Join the community of 1 Lakh+ Developers
Create a free account and get access to tutorials, jobs, hackathons, developer events and neatly written articles.
Create a free account