C Programming variables (Set 2)
MCQ problems on C Programming variables declarations and initializations.
1. |
Which is correct syntax to get remainder after dividing 3.14 by 2.1 ? |
A. |
remainder = 3.14 % 2.1; |
B. |
remainder = modf(3.14, 2.1); |
C. |
remainder = fmod(3.14, 2.1); |
D. |
Remainder cannot be obtained in floating point division. |
2. |
Types of linkage in C: |
A. |
Internal and External |
B. |
External, Internal and None |
C. |
External and None |
D. |
Internal |
3. |
Which special character is allowed in variable name ? |
A. |
* (asterisk) |
B. |
| (pipeline) |
C. |
_ (underscore) |
D. |
- (hyphen) |
4. |
How are the following statements related ?
|
A. |
Both are same |
B. |
Both are same but extern int func(); might be in another file |
C. |
int func(); will be override by extern int func(); |
D. |
None of the options |
5. |
Which is correct way to round off 3.77 to 4.0 ? |
A. |
ceil(3.77) |
B. |
floor(3.77) |
C. |
roundup(3.77) |
D. |
roundto(3.77) |
6. |
Which is appropriate data type in C programming to represent real number ? |
A. |
float |
B. |
double |
C. |
long double |
D. |
long long double |
7. |
Identify the data type that are/is NOT user defined: 1.
2.
3.
|
A. |
1 |
B. |
2 |
C. |
3 |
D. |
1 and 2 |
8. |
What can you say about following line of code:
|
A. |
It is declaring variable i |
B. |
It is defining variable i |
C. |
It will throw error |
D. |
It's a function. |
9. |
Identify declaration statement(s): 1.
2.
3.
|
A. |
1 |
B. |
2 |
C. |
1 and 3 |
D. |
3 |
10. |
Take a look at following piece of code:
What can you say about definition and declaration of variable x ? |
A. |
extern int x is declaration, int x = 24 is the definition |
B. |
int x = 24 is declaration, extern int x is the definition |
C. |
int x = 24 is definition, x is not defined |
D. |
x is declared, x is not defined |
11. |
Prototype of a function is mentioned during ____ |
A. |
Definition |
B. |
Declaration |
C. |
Prototyping |
D. |
Call |
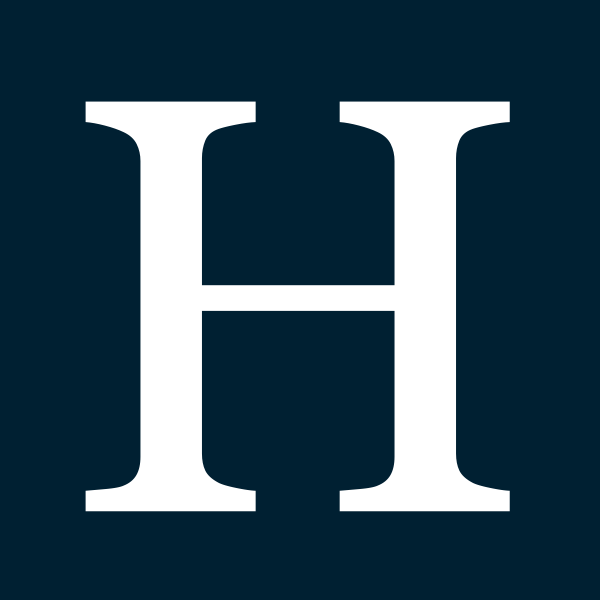
Join the community of 1 Lakh+ Developers
Create a free account and get access to tutorials, jobs, hackathons, developer events and neatly written articles.
Create a free account