FastAPI - Build your first API using FastAPI
Create a main.py file
After installation of FastAPI on your system Let's build a simple API using FastAPI. Open your favorite code editor and create a main.py file and paste following code:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def home():
return {"message": "Hello HackersFriend"}
Run your code
Now run your API using following command:
uvicorn main:app --reload
It should output on terminal like following:
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
INFO: Started reloader process [28720]
INFO: Started server process [28722]
INFO: Waiting for application startup.
INFO: Application startup complete.
Now visit http://127.0.0.1:8000/
You should see a response like following:
{"message":"Hello HackersFriend"}
Congratulations you built your first api using FastAPI!
FastAPI auto generated interactive API docs
FastAPI generates documentation for API as well.
Goto http://127.0.0.1:8000/docs to see the docs generate by FastAPI using Swagger UI. It should look like following:
FastAPI also generates alternative documentation using Redoc. To view these docs visit http://127.0.0.1:8000/redoc
It should look like following:
FastAPI also generates an OpenAPI compatible json output, which contains all of your API and their details.
To view that JSON output go to : http://127.0.0.1:8000/openapi.json
It should produce an output like following:
{"openapi":"3.0.2","info":{"title":"FastAPI","version":"0.1.0"},"paths":{"/":{"get":{"summary":"Home","operationId":"home__get","responses":{"200":{"description":"Successful Response","content":{"application/json":{"schema":{}}}}}}}}}
What can you do with OpenAPI JSON schema
The above OpenAPI schema is used by Swagger and Redoc to produce those 2 auto generated docs, that we just saw. Alternatively, if you want to use some other tools to generate your documentation, you can use this OpoenAPI schema. Most of the API doc generators usage OpenAPI schema to generate documentation. Other use case could be, you can use tools to generate code automatically for client side to communicate with your API end points. These can be frontends, mobile devices, etc.
Understand your code step by step
Now let's understand what we just did, step by step. We'll break it down line by line.
1. Import FastAPI:
from fastapi import FastAPI
FastAPI is a python class here. It provides all the functionalities that we need to implement our API, going forward we will create an instance of this class and that instance should have all the features that we need.
2. Create an instance of FastAPI
app = FastAPI()
Here we created an variable 'app' which is an instance of FastAPI class, we will use this instance to implement our API. This is the main interaction point of application.
Notice that, this is the same variable name that we passed with uvicorn while running our API.
> uvicorn main:app --reload
if we would have given this variable name to be 'hackersfriend' like following:
from fastapi import FastAPI
hackersfriend = FastAPI()
@hackersfriend.get("/")
def home():
return {"message": "Hello HackersFriend"}
we would be running it like following:
uvicorn main:hackersfriend --reload
3. Define functions for URL paths as per HTTP method
Which command is used to run FastAPI application ?
A. uvicorn main:app --reload
B. python main.py runserver
C. python main.py --port 8000
D. None of the options
See answer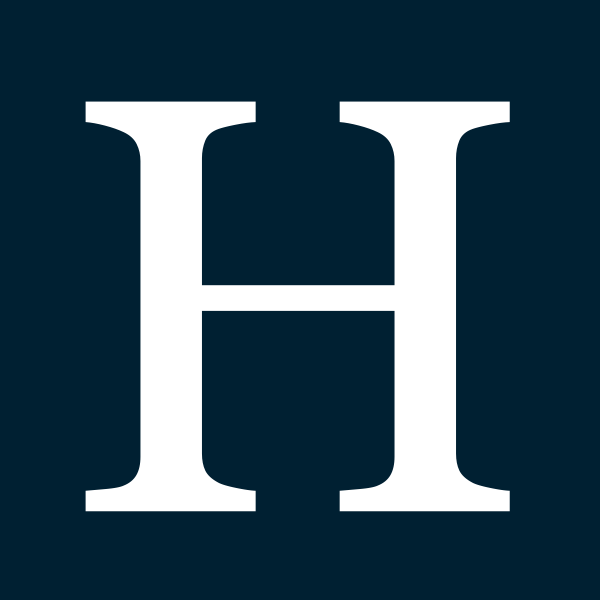
Join the community of 1 Lakh+ Developers
Create a free account and get access to tutorials, jobs, hackathons, developer events and neatly written articles.
Create a free account